Step 2: Customize the Payment Form
Merchants can configure the PayNearMe JS Library via a required initialization script (i.e., PMN.init
) that can include custom targets (modal, iframe, drawer, etc.), language preferences, and preloaded consumer data. Place this script reference after loading the JS library.
The following code displays a sample script.
<script>
PNM.init( {
"data": {
"disbursement_balance_amount": "4000.00",
"min_disbursement_amount": "25.00",
"max_disbursement_amount": "5000.00"
},
"auto_resize": true,
"language": "en",
"header_size": "35",
"header_bkgnd": "#385723",
"header_color": "ffffff",
"callback": "<YOUR_CALLBACK_FUNCTION>",
"target": "<YOUR_CONTAINER_ID>",
"actions": {
"<YOUR_PAYMENT_BUTTON_ID>": {
"action": "pay",
"header": "Make a Payment",
"debit": true,
"credit": true,
"ach": true,
"google_pay": true,
"cash": true,
"segmentation_id": "550e8400-e29b-41d4-a716-446655440000"
},
"<YOUR_DISBURSEMENT_BUTTON_ID>": {
"action": "withdrawal",
"header": "Disburse",
"debit": true,
"segmentation_id": "550e8400-e29b-41d4-a716-446655440000"
}
},
"header": "",
"order_token": "<YOUR_TOKEN>"
});
</script>
This script renders the following payment and disbursement screen (when the consumer clicks the configured pay or disburse buttons/links).
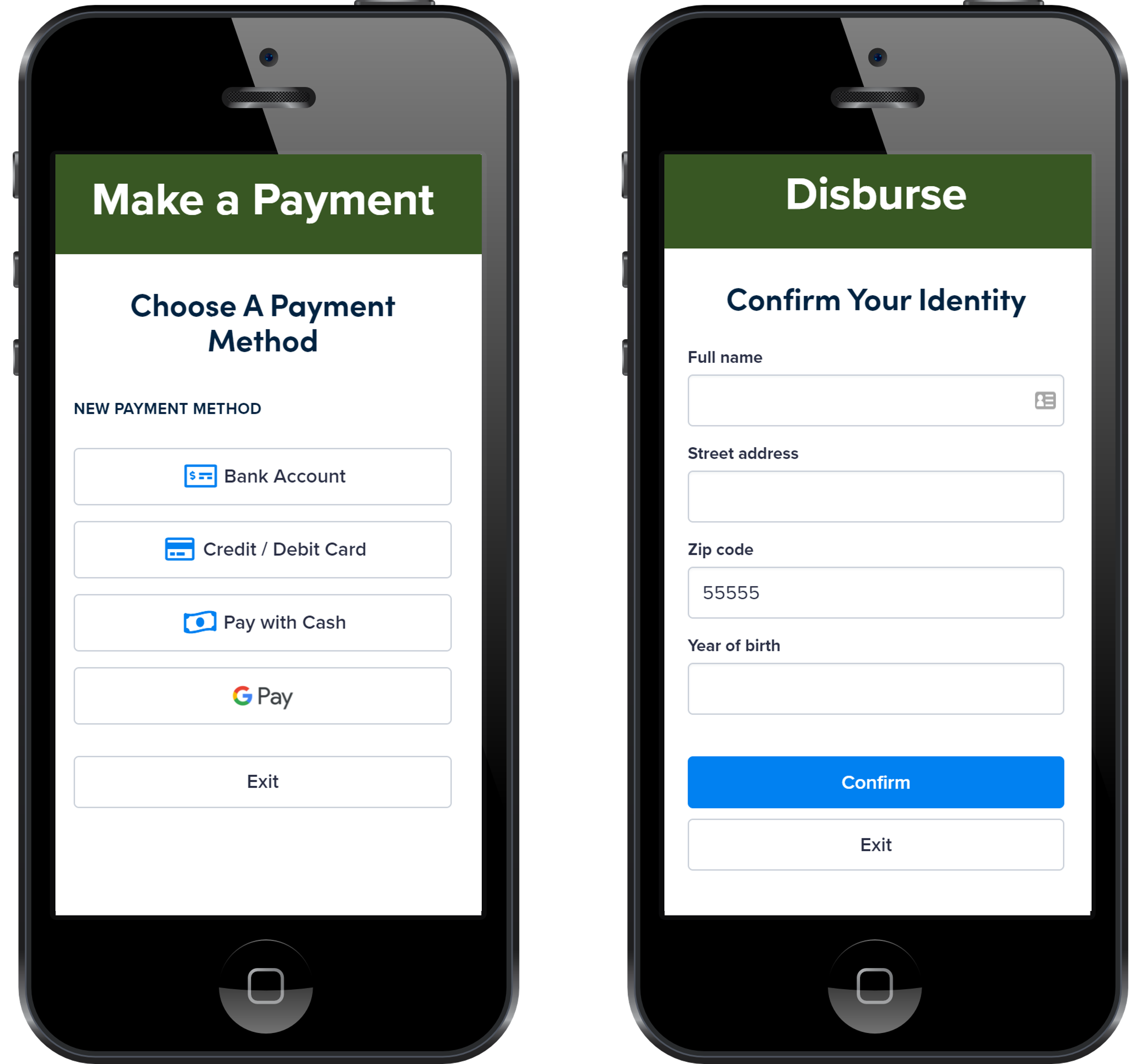
Screen After Clicking Payment Button (left) and Screen After Clicking Disburse Button (right)
Use the following parameters to configure the embedded client form.
Name | Description | Type | Required? |
---|---|---|---|
order_token | The secure_smart_token value that’s returned in the create_order and get_smart_token calls. This field is required and must be included to initialize the JS library. | string | R |
actions | An array of UI and button settings, including payment methods and behavior, defined by the given button ID. | array | R |
action | Defines the action(s) this button will perform on the order. Supported options include the following:
| enum | R |
header | The header text that displays centered at the top of the browser window. Headers can be defined per action or can be defined globally and display for all actions. | string | O |
ach | Use either true or false to define which payment types should be preloaded. | bool | O |
apple_pay | Use either true or false to define which payment types should be preloaded. NOTE: The Apple Pay option only displays on the Safari browser. When the consumer attempts to use Apple Pay from a browser that is not Safari, an error message displays. | bool | O |
cash | Use either true or false to define which payment types should be preloaded. | bool | O |
cash_app | Use either true or false to define which payment types should be preloaded. | bool | O |
credit | Use either true or false to define which payment types should be preloaded. | bool | O |
debit | Use either true or false to define which payment types should be preloaded. | bool | O |
google_pay | Use either true or false to define which payment types should be preloaded. | bool | O |
paypal | Use either true or false to define which payment types should be preloaded. | bool | O |
venmo | Use either true or false to define which payment types should be preloaded. | bool | O |
segmentation_id | A unique string ID (e.g., UUID). This field can be used for data analytics and reporting. | string | O |
hide_saved_payment_methods | When set to true , this setting hides existing, previously tokenized payment methods for all actions. When set to false , this setting shows all previously tokenized payment methods for all actions. When unset or null, the Embedded Client will hide saved payment methods for the tokenize action and show saved payment methods for the pay and withdrawal actions. | bool | O |
require_push_enabled | When set to true , this setting hides saved payment methods and rejects adding new payment methods that are not enabled for disbursements. NOTE: This setting applies only to the tokenize action. Use this setting when you create a standard order in advance, but want to use it only for disbursements when sending a user through the tokenize flow. | bool | O |
payment_amount | Use this parameter to force a fixed payment/disbursement amount. | dec | O |
payment_field_fixed | Set to true to prevent consumers from changing the amount. Setting this field to false creates an editable field where consumers can enter their own payment/disbursement amounts. | bool | O |
callback | The method to call when certain events occur, such as successful or declined payments or an error. This field is required. | func | R |
auto_resize | Setting this parameter to true ensures that the client remains responsive and will properly display in the HTML target as content is added and updated. | bool | O |
data | Use this object to define the consumer’s global disbursement limits. | obj | O |
data.max_disbursement_amount | The maximum allowable amount for a disbursement. This parameter can also be defined per action. For example, if your maximum allowable disbursement amount for ACH transactions was higher than other payment methods, you would include this parameter in an “ACH Disbursement” action that was separate from other disbursement actions. | string | O |
data.min_disbursement_amount | The minimum amount required for a disbursement transaction. This parameter can be defined per action. | string | O |
data.disbursement_balance_amount | The amount available for a disbursement. This parameter can be defined per action. | string | O |
language | Supported values for this field include the following:
| enum | O |
header_size | The font size of the header text in pixels. This parameter can be defined per action. | string | O |
header_bkgnd | The hex code of the header’s background color. If not defined, the embedded client defaults the color to Vivid Blue or #0181F1 . This parameter can be defined per action. | string | O |
header_color | The hex code of the header’s text. If not defined, the embedded client defaults the color to White or #ffffff . This parameter can be defined per action. | string | O |
target | The element where the iframe will display. Supported options include the following:
| string | O |
Updated 5 months ago