Integrating with the Web/JS Library
PayNearMe’s JS library can be implemented in four easy steps with minimal API interaction. After generating a Smart Token via the /create_order or /get_smart_token API calls, add it to the library’s initialization script (PNM.init
) along with any UI/UX customizations for the payment form. Use a button or link to invoke the JS library and the payment form in either a modal, drawer, or a DOM element where you want to insert the iframe. As the player enters his/her data, the payment form sends real-time callbacks to a JS method you define when certain events, like errors, timeouts, or canceled payments occur. After the player submits his/her data, the transaction is processed, and, if configured, PayNearMe sends Authorization and Confirmation callbacks to your server. You can configure the client to display in-app receipt language or redirect the app to a payment confirmation page you control.
Customizing the UI of the Embedded Client
The embedded client gives you the option to customize the UI of your payment form with brand-specific colors for the header, icons, and buttons, and the size and type of font for text. You can also choose to display available payment types as buttons or a clickable list.
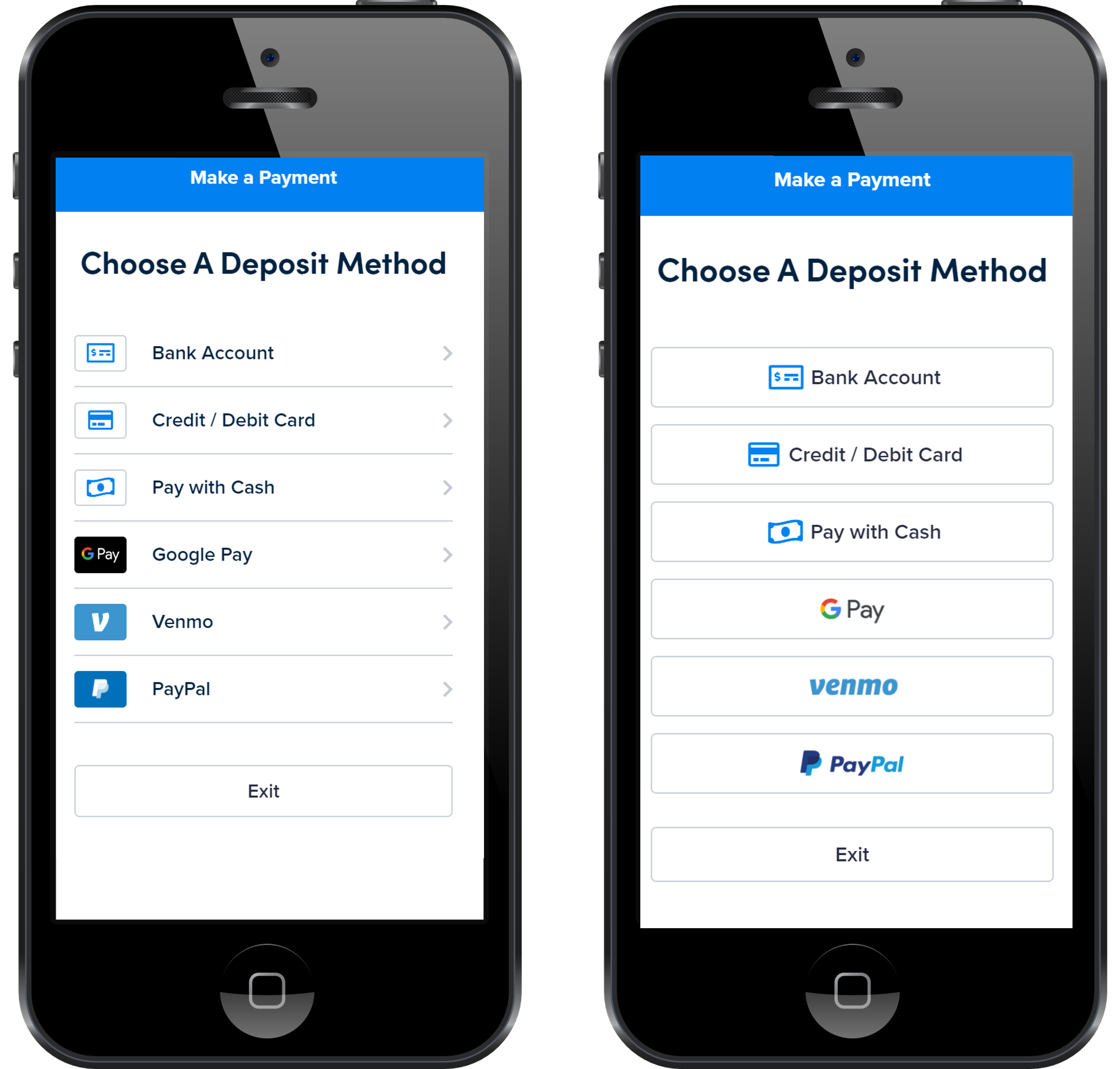
List View (left) and Button View (right)
For more information on customizations, contact your Implementation Specialist.
Integration Use Cases
PayNearMe for iGaming uses a modal, drawer, or
- Capture deposits
- Initiate withdrawals
- Tokenize payment methods
Each form is generated from a session-timed script with encrypted, tokenized data values that authenticate genuine PayNearMe orders. Operators can define how many times players can access the form and for how long, and once accessed, how long the form will display until expiration. This protects sensitive player data and helps prevent cross-site request forgery attacks. For troubleshooting and data analytics, the embedded client can provide real-time event descriptions through client-side callbacks to your server.
For direct customer engagement, PayNearMe’s Smart Link™ gives players the ability to complete deposits with just a few clicks. Operators can customize the Smart Link URL
- to only display Operator-specified payment types,
- to display redirect URLs for specific scenarios like errors and abandoned payments, and/or
- to expire after a specified amount of time has passed.
For security, PayNearMe encrypts order information and link customizations within the Smart Token that is appended to the Smart Link. This token authenticates the order and preloads player information into the form, making the deposit process easier and more secure.
The following sections provide details on how to connect your system to PayNearMe.
Use Case: Capturing Deposits
The payment process for electronic deposits only requires one API call from the Operator. PayNearMe handles all data input and processing, so players can quickly deposit funds and begin placing their bets. The following diagram displays a simplified dataflow of events for electronic deposits (e.g., non-cash).
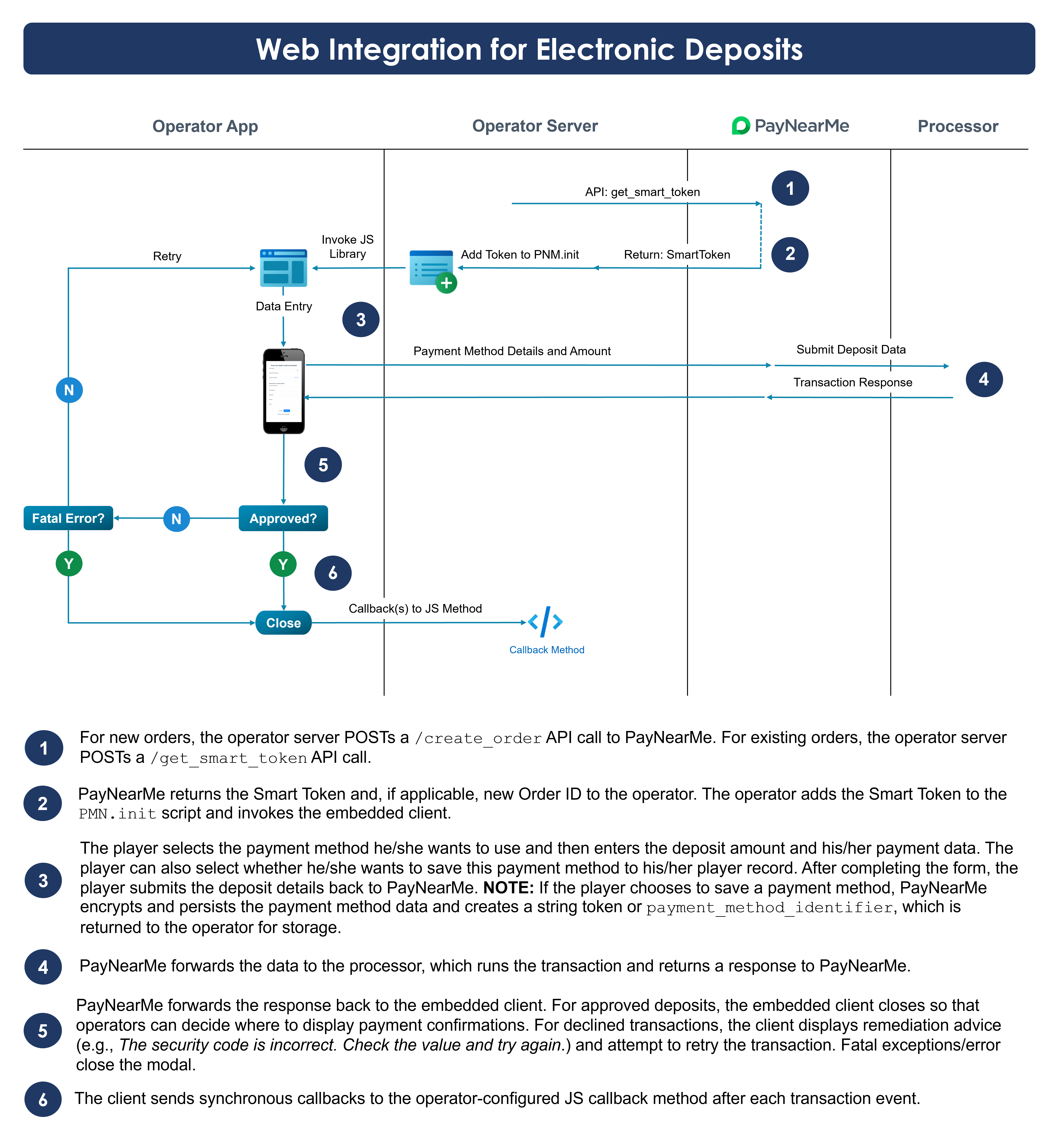
Cash deposits initiated in the embedded client are set up using the same methods; however, the flow requires a few extra steps. The following diagram displays the cash deposit flow.
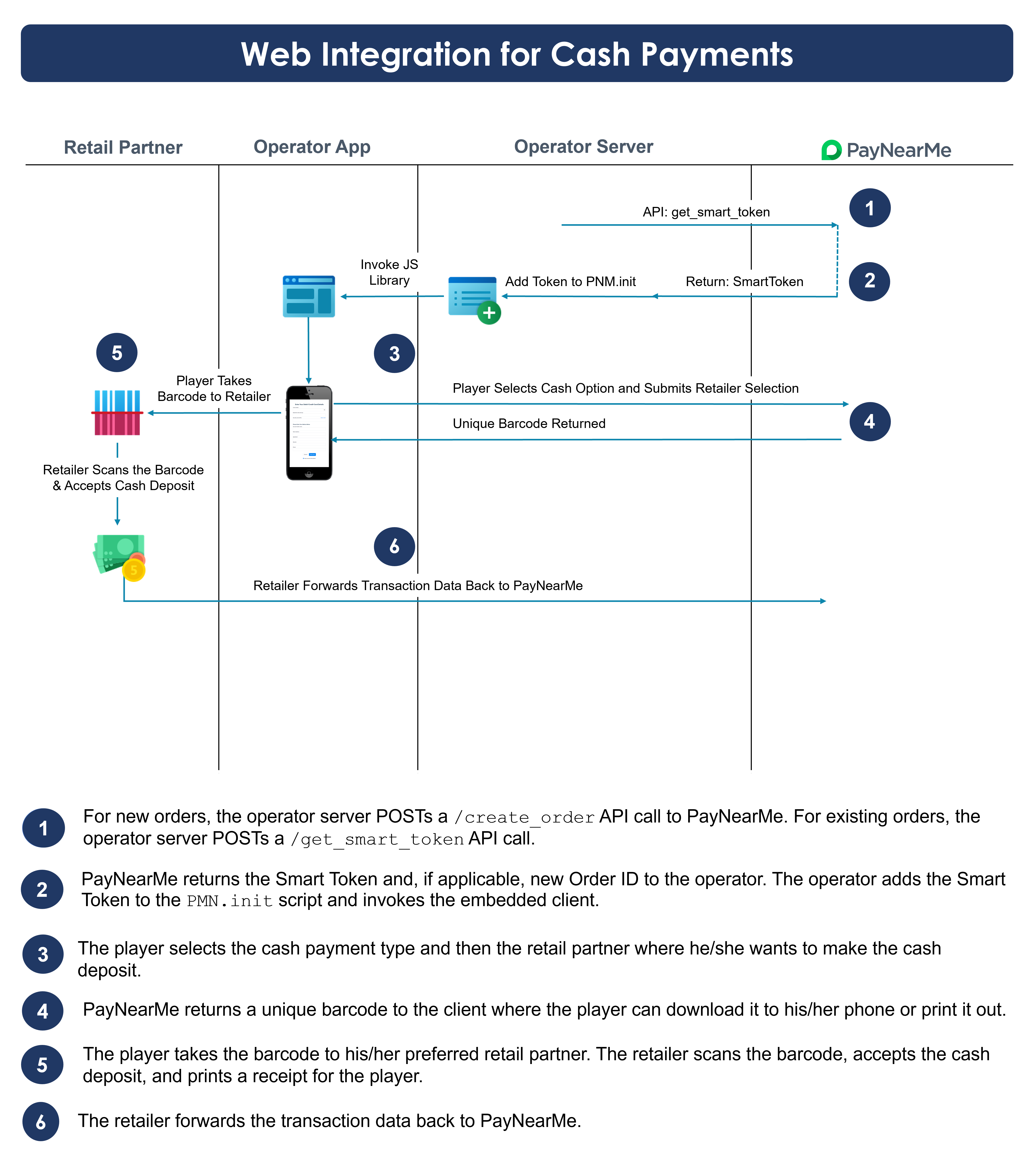
Use Case: Initiating Withdrawals
The withdrawal process also requires only one API call from the Operator (get_smart_token
). Withdrawals require an existing Order ID for the player and, if configured, a saved payment method that can accept withdrawal funds. Payment method requirements depend on your specific site settings. To allow players to create payment methods at the time of withdrawal, consult with your Implementation Specialist about your site’s configuration. Additionally, withdrawals require identity and compliance checks prior to money disbursement (e.g., funds cannot be transmitted to players on the OFAC Sanctions List). The following diagram displays the withdrawal process.
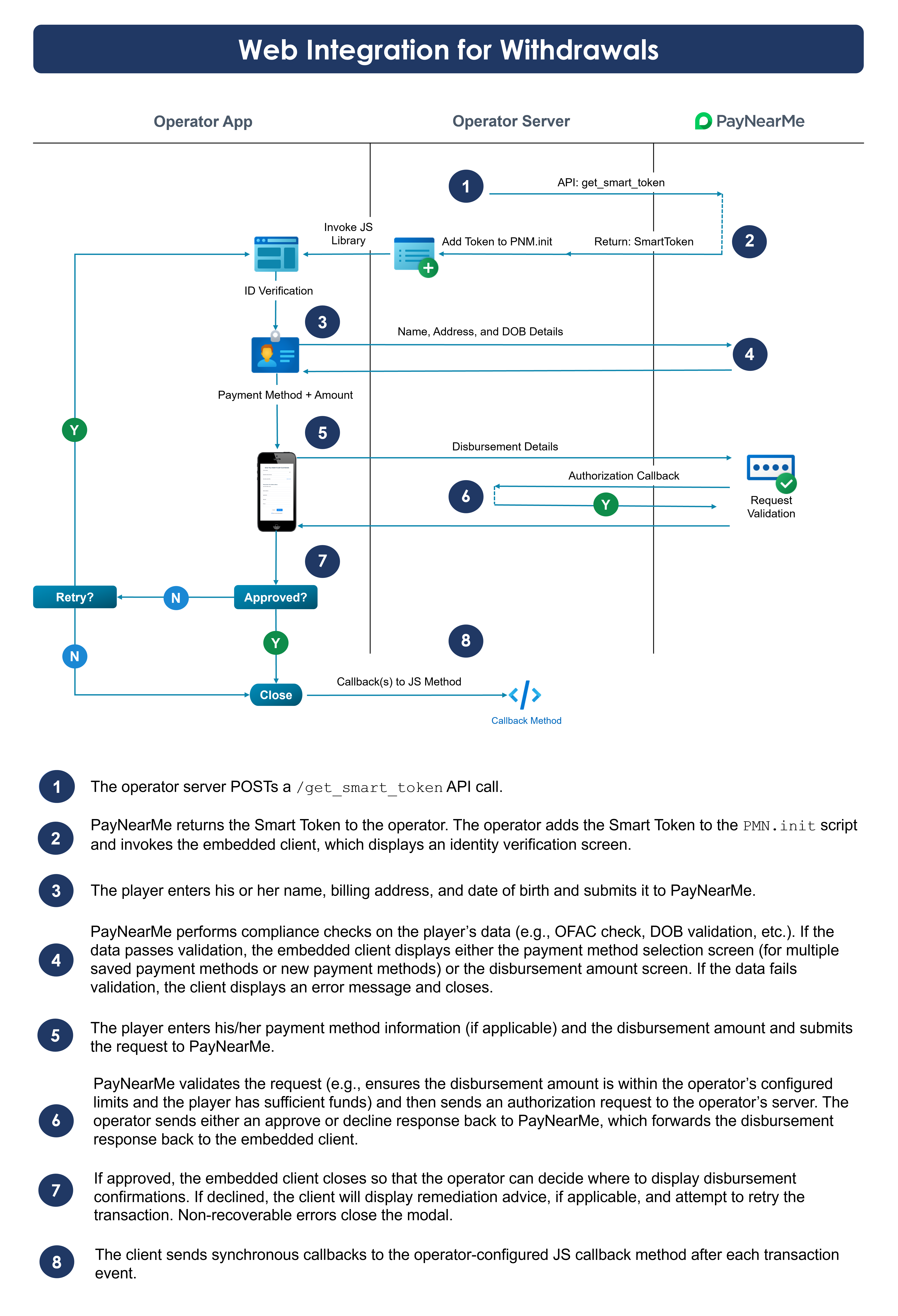
Use Case: Tokenizing Payment Methods
PayNearMe’s tokenization solution reduces your PCI scope and protects sensitive player data from bad actors. Giving players the ability to quickly add and save payment types they can use again and again makes both the deposit and withdrawal process easier and more efficient. This solution is for Operators who already have a payment processor, but need a tokenization solution for safe, compliant payment data storage. The following diagram provides a basic flow of events for using PayNearMe’s tokenization solution.
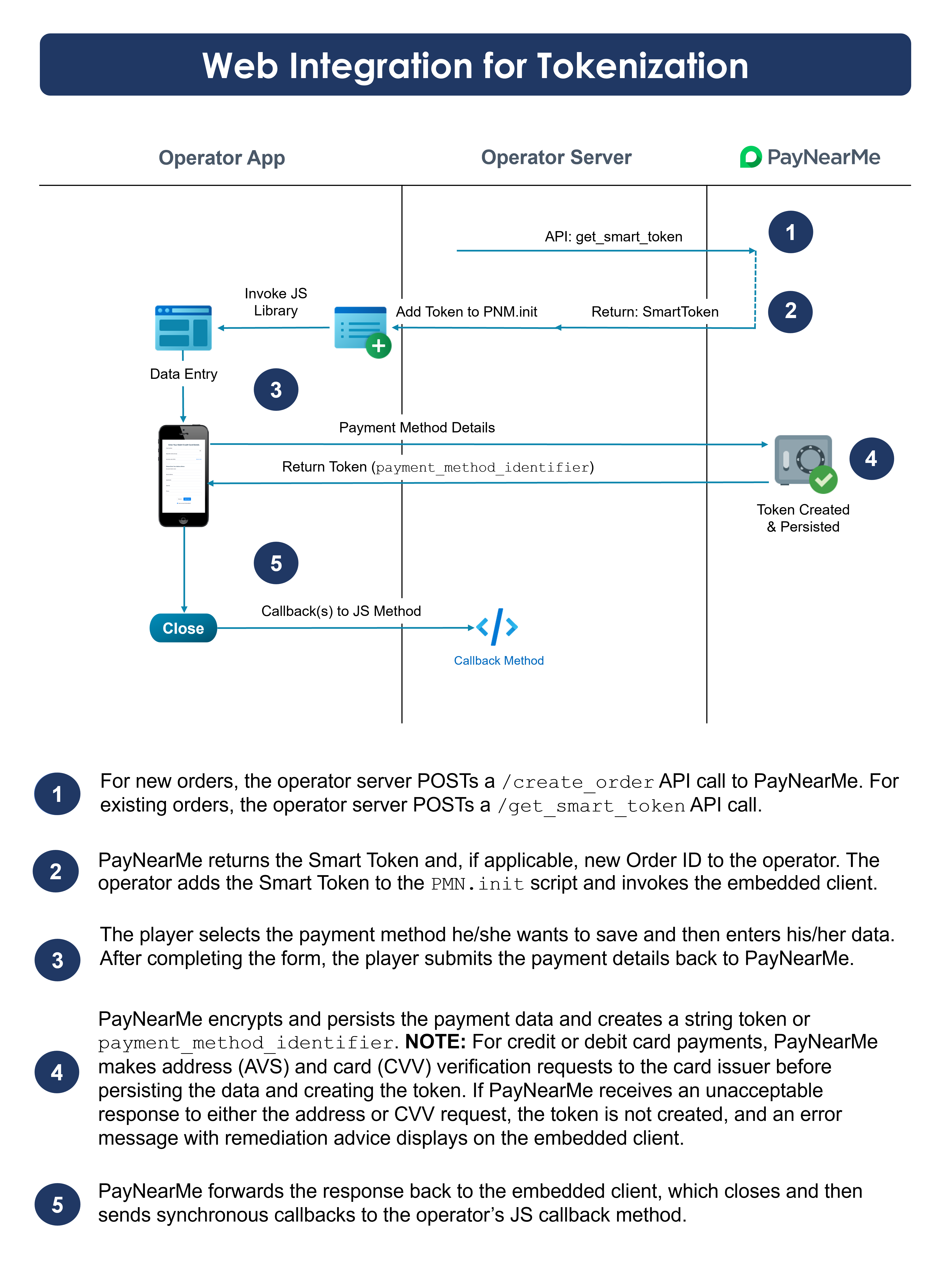
Setting Up Your Cashier Page
When configuring your Cashier page, perform the following tasks to use PayNearMe’s embedded client:
Load the PayNearMe JS Library.
- Initialize the PayNearMe JS Library with your Smart Token and any customizations.
- Include the Make Deposit button or link (or any clickable HTML tag) that invokes the PayNearMe JS Library.
- Include a callback script that defines how the iframe should behave when specific events occur (e.g., success, failure, error, etc.).
Which Callback?
The client-side callback is different from the server-side Authorization, Confirmation, Reversal, and Order Change callbacks. See the Callbacks page for more information.
Load the PayNearMe JS Library
The PayNearMe JS Library initiates the embedded client, which is hosted and managed by PayNearMe. The client is displayed in either a named DOM element that you define or in a modal (overlay or side-drawer). Add this script to the bottom of your Cashier page in the applicable environments to load the JS Library:
- Sandbox:
<script src='https://www.paynearme-sandbox.com/api/cf/<site_identifier>/v1/ paynearme.js’></script>
- Production:
<script src='https://www.paynearme.com/api/cf/<site_identifier>/v1/paynearme.js’></script>
Configure and Initialize the JS Library
Operators can configure the PayNearMe JS Library via a required initialization script (i.e., PMN.init
) that can include custom targets (modal, iframe, drawer, etc.), language preferences, and preloaded player data. Place this script reference after loading the JS library.
The following code displays a sample script:
<script>
PNM.init( {
"data": {
"disbursement_balance_amount": "4000.00",
"min_disbursement_amount": "25.00",
"max_disbursement_amount": "5000.00"
},
"auto_resize": true,
"language": "en",
"header_size": "35",
"header_bkgnd": "#581845",
"header_color": "ffffff",
"callback": "<YOUR_CALLBACK_FUNCTION>",
"target": "<YOUR_CONTAINER_ID>",
"actions": {
"<YOUR_DEPOSIT_BUTTON_ID>": {
"action": "pay",
"header": "Deposit",
"debit": true,
"credit": true,
"ach": true,
"apple_pay": true,
"google_pay": true,
"paypal": true,
"venmo": true,
"cash": true
},
"<YOUR_WITHDRAWAL_BUTTON_ID>": {
"action": "withdrawal",
"header": "Withdraw",
"debit": true
}
},
"header": "",
"order_token": "<YOUR_TOKEN>"
});
</script>
Use the following parameters to configure the embedded client form:
Name | Description | Type | Required? |
---|---|---|---|
order_token | The secure_smart_token value that’s returned in the /create_order and /get_smart_token call. This field is required and must be included to initialize the JS library. | int | R |
actions | An array of UI and button settings, including payment methods and behavior, defined by the given button ID. | array | R |
action | Defines the action(s) this button will perform on the order. Supported options include the following:
| string | R |
header | The header text that displays centered at the top of the browser window. Headers can be defined per action or can be defined globally and display for all actions. | string | O |
hide_saved_payment_methods | When set to true , this setting hides existing, previously tokenized payment methods for all actions. When set to false , this setting shows all previously tokenized payment methods for all actions. When unset or nil, the Embedded Client will hide saved payment methods for the tokenize action and show saved payment methods for the pay and withdrawal actions. | bool | O |
require_push_enabled | When set to true , this setting hides saved payment methods and rejects adding new payment methods that are not enabled for withdrawals. NOTE: This setting applies only to the tokenize action. Use this setting when you create a standard order in advance but want to use it only for withdrawals when sending a user through the tokenize flow. | bool | O |
payment_amount | Use this parameter to force a fixed deposit/withdrawal amount. | num | O |
payment_field_fixed | Set to true to prevent players from changing the amount. Setting this field to false creates an editable field where players can enter their own deposit/withdrawal amounts. | bool | O |
debit | Use either true or false to define whether or not to preload this payment method. | bool | O |
credit | Use either true or false to define whether or not to preload this payment method. | bool | O |
ach | Use either true or false to define whether or not to preload this payment method. | bool | O |
cash | Use either true or false to define whether or not to preload this payment method. | bool | O |
apple_pay | Use either true or false to define whether or not to preload this payment method. NOTE: The Apple Pay option only displays on the Safari browser. When the player attempts to use Apple Pay from a browser that is not Safari, an error message displays. | bool | O |
google_pay | Use either true or false to define whether or not to preload this payment method. | bool | O |
paypal | Use either true or false to define whether or not to preload this payment method. | bool | O |
venmo | Use either true or false to define whether or not to preload this payment method. | bool | O |
cashapp | Use either true or false to define whether or not to preload this payment method. | bool | O |
callback | The method to call when certain events occur, such as successful or declined deposits or an error. This field is required if not using a redirect. | func | R |
auto_resize | Setting this parameter to true ensures that the client remains responsive and will properly display in the HTML target as content is added and updated. | bool | O |
data | Use this object to define the player’s global withdrawal limits. | obj | O |
data.max_disbursement_amount | The maximum allowable amount for a withdrawal. This parameter can also be defined per action. For example, if your maximum allowable withdrawal amount for ACH transactions was higher than other payment methods, you would include this parameter in an “ACH Withdrawal” action that was separate from other withdrawal actions. | string | O |
data.min_disbursement_amount | The minimum amount required for a withdrawal transaction. This parameter can be defined per action. | string | O |
data.disbursement_balance_amount | The amount available for a withdrawal. This parameter can be defined per action. | string | O |
language | Supported values for this field include the following:
| string | O |
header_size | The font size of the header text in pixels. This parameter can be defined per action. | string | O |
header_bkgnd | The hex code of the header’s background color. If not defined, the embedded client defaults the color to Vivid Blue or #0181F1. This parameter can be defined per action. | string | O |
header_color | The hex code of the header’s text. If not defined, the embedded client defaults the color to white or #ffffff. This parameter can be defined per action. | string | O |
target | The element where the iframe will display. Supported options include the following:
| string | O |
Including the Button
Any clickable function can be used to invoke the PayNearMe JS library and pass in the configured parameters of PMN.init
. PayNearMe’s code can connect to click handler functions on your page once the ID of the clickable element is set to the name of the action defined in the PMN.init
script. The following code sample display these configurable named actions:
"actions": {
"Deposit": {
"action": "pay",
"debit": true,
"credit": true,
"ach": true,
"apple_pay": true,
"google_pay": true,
"paypal": true,
"venmo": true,
"cash": true
},
"Withdrawal": {
"action": "withdrawal",
"debit": true
},
"Add_Card": {
"action": "tokenize",
"debit": true,
"credit": true
},
"Add_Bank_Account": {
"action": "tokenize",
"ach": true
}
}
Alternatively, you can manually call PNM.launch('name')
where ‘name’
is the name of the action defined in PMN.init
(e.g., PNM.launch(‘Deposit’)
).
The Client-Side Events Callback
Operators must create a callback function that the JS Library references in the callback field (e.g., callback: mycallbackfunction)
. Use the console.log()
function when creating your callback to record events and capture alert messages in real-time. This callback function should be placed before PNM.init
on your Cashier page and should provide guidance on how to handle errors, timeouts, and/or specific alert messages. The following is a sample of what your callback script may look like:
<script>
function mycallbackfunction(data){
console.log('mycallbackfunction',data);
if( !data.status ){
// status should always be present
// for any messages posted by the PNM iFrame.
console.log('[mycallbackfunction] Missing expected field');
return;
}
if( data.status == 'error' ){
// Operators may want to branch off depending on the error.
}
if( data.status == ‘exit’ ){
// The user exited the PNM UI flow before completion.
// Add code to guide the user as needed by your system.
PNM.close_modal();
}
if( data.status == 'complete' ){
PNM.close_modal();
// Add code to save off the response information to your system
}
msg = "Status: "+data.status + "\n";
if( data.message ){
msg += data.message.join("\n");
}
alert( msg );
}
</script>
PNM Response Data
Parameter | Description | Type | Always Included? |
---|---|---|---|
status | The status of the payment, withdrawal, or tokenization attempt. The following options are supported:
| string | Y |
message | An array of user-friendly, localized (English or Spanish) strings that describe the event. Multiple lines are broken into separate rows of the array and can be joined together using your preferred line-break method. Lines that have key-value pairs are listed in a single string separated by two pipe characters. For example, " For example, "Payment Amount||$101.23". | array | Y |
payment | The Payment Object. | JSON obj | N |
payment.payment_made | The date and time when the transaction occurred in Epoch time. | string | N |
payment.payment_amount | The decimal value of the payment amount (e.g., 104.99) | num | N |
payment.payment_currency | The currency code of the payment amount. Currently, only USD is accepted. | enum | N |
payment.payment_status | The status of the transaction. The following values are supported:
| string | N |
payment.payment_type | The type of payment method. Supported values include the following:
| string | N |
payment.payment_account | A short description of the payment method. | string | N |
payment.net_payment_amount | The payment amount that is settled to the customer after all merchant, retailer (if applicable), and PayNearMe fees have been taken out. | num | N |
payment.net_payment_currency | The currency code of the net payment amount. Currently, only USD is accepted. | enum | N |
payment.payment_processing_fee | The fee amount that PayNearMe charges for processing the transaction | num | N |
payment.due_to_parent | The amount settled to the parent site when set up in a parent/child site relationship. NOTE: This field applies only to ISV integrations. | string | N |
payment.due_to_parent_currency | The currency code of the amount due to the parent site. Currently, only USD is accepted. NOTE: This field applies only to ISV integrations. | enum | N |
payment.due_to_child | The amount settled to the child site when set up in a parent/child site relationship. NOTE: This field applies only to ISV integrations. | string | N |
payment.due_to_child_currency | The currency code of the amount due to the child site. Currently, only USD is accepted. NOTE: This field applies only to ISV integrations. | enum | N |
payment.settled_to_site | Indicates whether the transaction funds have been settled to the merchant site. | bool | N |
payment.date_settled_to_merchant | The date and time when the transaction was settled to the merchant in Epoch time. | string | N |
payment.pnm_payment_identifier | A unique ID generated for each transaction | string | N |
payment.retailer_identifier | A unique PayNearMe-assigned ID for the retailer | string | N |
payment.merchant_settlements | An array of merchant settlements | array | N |
payment.merchant_settlements.settlement_method_identifier | A unique ID that identifies a settlement | string | N |
payment.merchant_settlements. settlement_type | Identifies whether the settlement is a net settlement or a gross settlement | string | N |
payment.merchant_settlements. settlement_amount | The amount to be settled | string | N |
payment.merchant_settlements.settlement_currency | The currency code of the settlement amount. Currently, only USD is accepted. | enum | N |
payment_method | The Payment Method object | JSON obj | N |
payment_method.type | The type of payment method. Supported values include the following:
| string | N |
payment_method.fee_amount | The service/convenience fee associated with this payment method type. | num | N |
payment_method.fee_currency | The currency code of the service/convenience fee amount. Currently, only USD is accepted. | enum | N |
payment_method.accounts | An array of payment method accounts | array | N |
payment_method.accounts.payment_method_identifier | The unique ID for this payment method | string | N |
payment_method.accounts.status | The status of this payment method. The following values are supported:
| string | N |
payment_method.accounts.name | The given name of this payment amount | string | N |
payment_method.accounts.description | A short description of this payment account (e.g., “Debit Card” or “Berkshire Ltd Bank”) | string | N |
payment_method.accounts.number | The last four digits of the card or bank account number | num | N |
payment_method.accounts.fee_amount | The service/convenience fee associated with this payment account | num | N |
payment_method.accounts.fee_currency | The currency code of the service/convenience fee amount. Currently, only USD is accepted. | enum | N |
last_response | The Last Response object. If the player exits the PayNearMe flow without completing the desired action, a “last_response” object will be included in the callback when the player exits. The object will contain information about the last significant action the player took before exiting. | JSON obj | N |
last_response.status | The status of the action when the user exited the PayNearMe flow. Supported values include the following:
| string | N |
last_response.response_code | The API Response Code | int | N |
last_response.message | A description of the events displayed in an array of strings | array | N |
auto_pay | The Autopay object. A future-scheduled payment (one-time or recurring) will have an auto_pay object instead of a payment object. | JSON obj | N |
auto_pay.schedule | A human-friendly description of the payment schedule. Displays for all schedules except those where frequency=once . | string | N |
auto_pay.frequency | Indicates how often a payment occurs in this autopay schedule. Supported values include the following:
| string | N |
auto_pay.amount | The amount of each scheduled payment without the service/convenience fees included. | num | N |
auto_pay.start_date | The date when the autopay schedule begins in YYYY-MM-DD format. | string | N |
auto_pay.duration_type | Defines how long this autopay schedule will run. Supported options include the following:
| string | N |
auto_pay.end_date | The date when the autopay schedule ends in YYYY-MM-DD format. | string | N |
auto_pay.scheduled_payments | The total number of payments that will be made for this schedule. This parameter is only used if duration_type=number_of_recurrences . | string | N |
Sample Deposit Success Callback
{
"status": "complete",
"code": 0,
"message": [
"Payment Complete",
"Payment Date||10/14/21",
"Account||198640000",
"Customer||Raymond Stantz",
"Confirmation number||234478391623",
"Payment Method||Credit Card 9999",
"Payment Amount||$100.00",
"Service Fee||$4.99",
"Total Payment||$104.99"
],
"payment_method":{
"payment_method_identifier":"8eb1948964b7b",
"type": "credit",
"status": "active",
"name": "Dr. Raymond Stantz",
"description": "Credit Card",
"account_type": "Credit",
"number": "9999",
"fee_amount": "4.99",
"fee_currency": "USD"
},
"payment": {
"payment_identifier": "786715791256",
"total_amount": "102.32",
"total_currency": "USD",
"payment_amount": "100.33",
"payment_currency": "USD"
}
}
Sample Withdrawal Success Callback
{
"status": "complete",
"code": 0,
"message":[
"Card load completed successfully from Hard Rock Casino",
"Amount loaded||$74.01",
"Processing fee||$0.99",
"Total amount||$75.00",
"Confirmation||913963157076"
],
"payment_method":{
"payment_method_identifier": "b31d6dc606f5e",
"type": "debit",
"status": "active",
"name": "Egon Spengler",
"description": "Debit Card",
"account_type": "Debit",
"number": "9997",
"fee_amount": "4.99",
"fee_currency": "USD"
},
"payment":{
"payment_made": "2021-10-14 14:47:38 -0700",
"payment_amount": "75.00",
"payment_currency": "USD",
"payment_status": "approved"
}
}
Sample Tokenize Success Callback
{
"status": "complete",
"code": 0,
"message": [
"Payment Method added successfully"
],
payment_method: {
"payment_method_identifier": "ob3e46a4eee9c",
"type": "ach",
"status": "active",
"name": "Dana Bennett",
"description": "Star City Federal Credit Union",
"account_type": "Personal Checking",
"number": "8103",
"fee_amount": "4.99"
"fee_currency": "USD"
}
}
Sample Authorized Success Callback
{
"status": "authorized",
"message":
[
"Payment Authorized",
"Payment Date||1/11/22",
"Account||123456789",
"Customer||Patsy",
"Confirmation Number||592104711367",
"Payment Method||Cash at 7-Eleven",
"Payment Amount||$250.00",
"Service Fee||$2.99",
"Total Payment||$252.99",
"A confirmation has been sent to (555) 500-0002 and [email protected]"
],
"payment_method":
{
"type": "cash",
"description": "Cash at 7-Eleven"
},
"payment":
{
"payment_made": "2022-01-11 08:00:00 -0800",
"payment_amount": "252.99",
"payment_currency": "USD",
"payment_status": "authorized",
"net_payment_amount": "250.00",
"net_payment_currency": "USD",
"payment_processing_fee": "2.99",
"payment_processing_fee_currency": "USD",
"pnm_processing_fee": "2.99",
"pnm_processing_fee_currency": "USD",
"settled_to_site": "false",
"date_settled_to_merchant": "",
"pnm_payment_identifier": "592104711367",
"retailer_identifier": "7-Eleven",
"site_channel": "consumer",
"payment_receipt_text": "Please try again in a minute to get your receipt.\n\n",
"payment_latitude": "26.3623",
"payment_longitude": "-80.0901",
"payment_location_identifier": "14338",
"payment_location_retailer": "7-Eleven",
"payment_location_address": "Holly Street, San Carlos, CA",
"merchant_settlements":
[
{
"settlement_method_identifier": "SM754042196",
"settlement_type": "net_payment",
"settlement_amount": "250.00",
"settlement_currency": "USD"
}
]
}
}
Exit After Error Callback
{
"status": "exit",
"message": [
"Action cancelled"
],
"last_response": {
"status": "error",
"response_code": "5000",
"message": [
"Minimum required payment is $20.00. Please retry transaction with this amount. You may contact your merchant or PayNearMe Support at 1-888-714-0004 for assistance."
]
}
Exit Before Deposit Completion Callback
{
"status": "exit",
"message": [
"Action Canceled"
],
"last_response": {
"message": [
"payment_method created"
],
"response_code": "0"
}
Updated 2 months ago